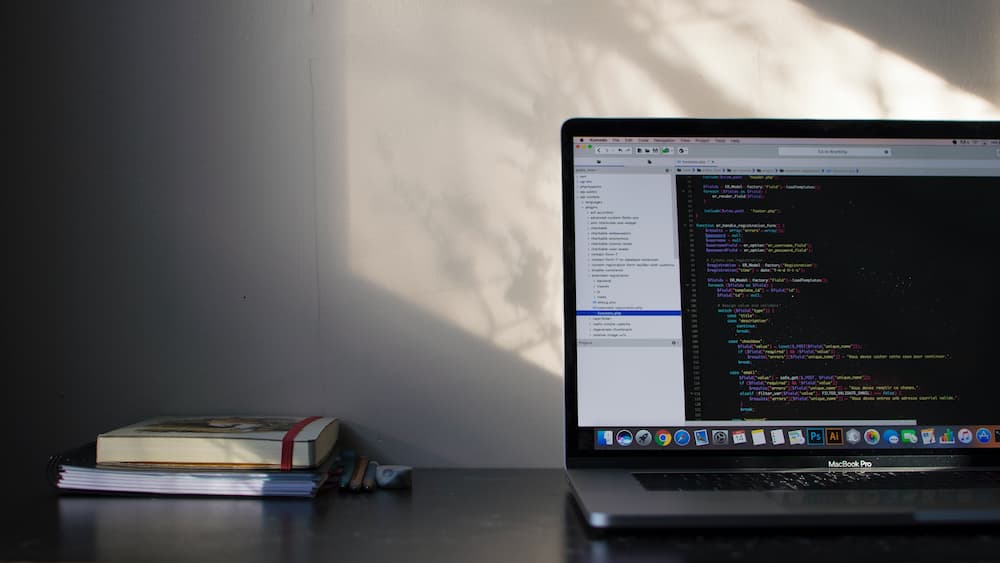
Structure:
Configuration (db.php – DB connection)
- Home page – index.php (login form)
- Teams (teams.php – a list of teams with the ability to edit team information)
- Players (players.php – a list of players with the ability to edit player information)
- Countries (countries.php – a list of teams by country)
In my projects, I use the generic PDO driver to work with the database. There are other options for working with databases, such as mysql and mysqli. Immediately, I note that the mysql extension is considered obsolete since php 5.5, and removed from version 7.
Why am I using PDO? Its main advantage is its versatility: PDO can work freely with different manufacturers of DBMS, which makes the transition from one DBMS to another from the point of view of php less expensive. We will consider further details and features of working with PDO in the next lessons, in the process of writing our application.
Also in this lesson you will learn about the try… catch construct. When is this design used? In the process of creating programs, errors occur (logic errors, typos, etc.), but errors may occur that you can initially foresee. For example, you are well aware that the connection to the database may end with an error, and this error must be foreseen.
Try … catch example
<?php
try {
$db = new PDO("mysql:host=localhost;dbname=sport", $user, $password);
} catch (Exception $e) {
echo $e->getMessage();
}
After successfully connecting to the database, we will write a skeleton for the appearance of the main page of our application using bootstrap and jquery.
Code (db.php)
<?php $user = "root"; $password = "29061990";
try {
$db = new PDO("mysql:host=localhost;dbname=sport", $user, $password);
} catch (Exception $e) {
echo $e->getMessage();
}
Code (index.php)
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Document</title>
<link href="//maxcdn.bootstrapcdn.com/bootstrap/3.3.6/css/bootstrap.min.css" rel="stylesheet">
<script src="https://code.jquery.com/jquery-3.1.1.min.js"></script>
<script src="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/js/bootstrap.min.js"></script>
</head>
<body>
<header>
<nav class="navbar navbar-default" role="navigation">
<div class="container-fluid">
<div class="navbar-header">
<button type="button" class="navbar-toggle" data-toggle="collapse" data-target=".navbar-ex1-collapse">
<span class="sr-only">Toggle navigation</span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
<span class="icon-bar"></span>
</button>
<a class="navbar-brand" href="#">Sport CRM</a>
</div>
<div class="collapse navbar-collapse navbar-ex1-collapse">
<ul class="nav navbar-nav">
<li><a href="index.php">Home</a></li>
<li><a href="players.php">Players</a></li>
<li><a href="teams.php">Teams</a></li>
<li><a href="countries.php">Countries</a></li>
</ul>
</div>
</div>
</nav>
</header>
<div id="content">
</div>
<footer>
</footer>
</body>
</html>