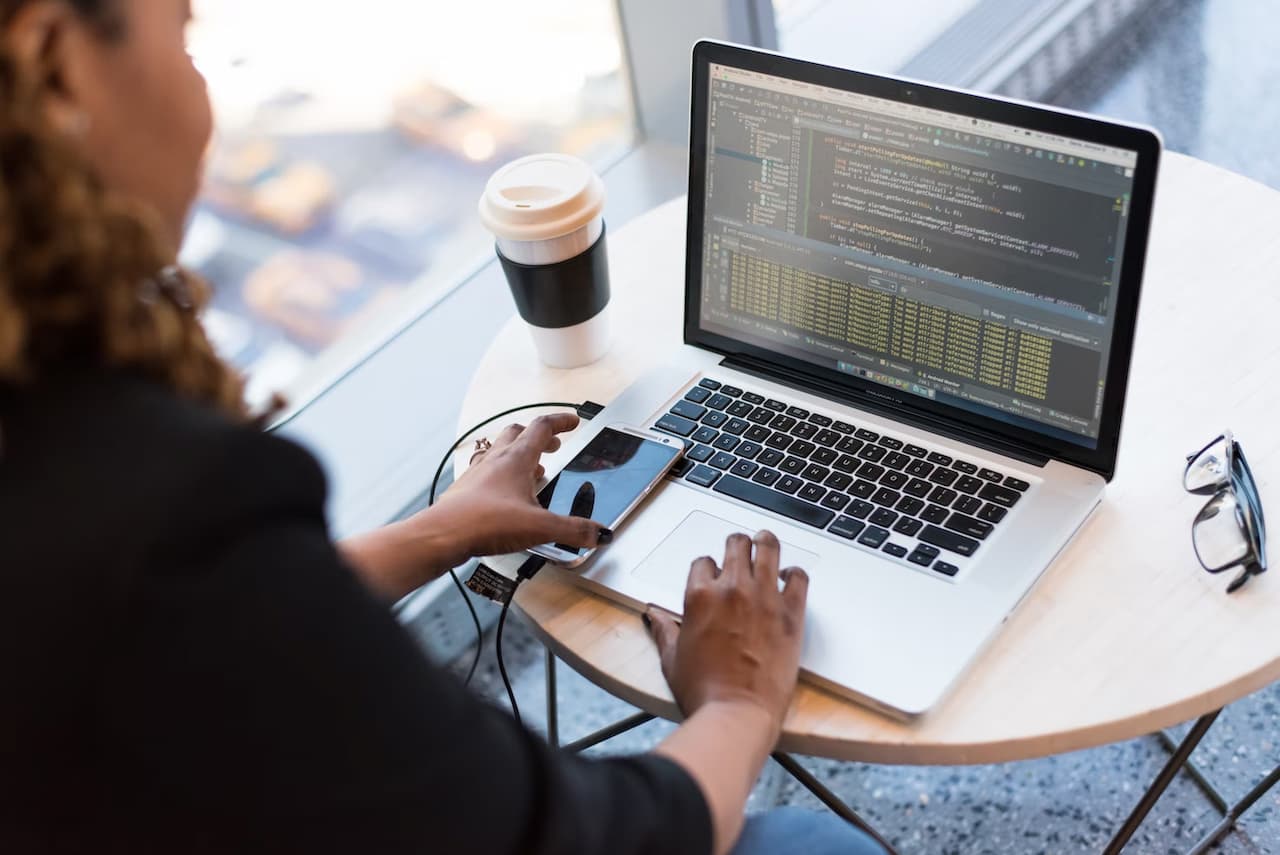
PHP is a server-side scripting language that is widely used for developing dynamic web pages and web applications. It is free, open-source, and supported by a large community of developers. In this beginner’s guide, we will cover the basics of PHP and how to get started with your first PHP project.
Installing PHP
Before you can start coding with PHP, you need to install it on your computer. PHP can be installed on various operating systems such as Windows, macOS, and Linux. The easiest way to install PHP is to use a web server such as Apache or Nginx, which has PHP pre-installed.
If you are using a Windows operating system, you can use WAMP or XAMPP, which is an all-in-one package that includes PHP, Apache, and MySQL. If you are using macOS, you can use MAMP, which is similar to WAMP or XAMPP. For Linux users, you can install PHP through the package manager.
Setting up a development environment
After installing PHP, you need a code editor or an integrated development environment (IDE) to write and edit your PHP code. There are many free and paid options available such as Visual Studio Code, Sublime Text, and PhpStorm.
Once you have your code editor installed, you can create a new file with a “.php” extension. This is the file extension that PHP recognizes, and it tells the web server to execute the code as PHP.
Your first PHP script
Now that you have your development environment set up, it’s time to write your first PHP script. In PHP, code is enclosed in “<?php” and “?>” tags. This tells the server that the code between the tags should be executed as PHP code.
Here’s an example of a simple PHP script that prints “Hello, World!” on the screen:
<?php
echo “Hello, World!”;
?>
In this script, we used the “echo” statement to output the string “Hello, World!” to the screen. The semicolon at the end of the statement indicates the end of the line.
Variables in PHP
Variables are used to store data in PHP. A variable is declared using the “$” sign, followed by the variable name. The value of the variable can be assigned using the “=” sign.
Here’s an example of a PHP script that declares a variable and assigns a value to it:
<?php
$message = “Hello, World!”;
echo $message;
?>
In this script, we declared a variable named “message” and assigned the string “Hello, World!” to it. We then used the “echo” statement to output the value of the variable to the screen.
Working with arrays
Arrays are used to store multiple values in PHP. An array is declared using square brackets “[]” and can contain any number of values.
Here’s an example of a PHP script that declares an array and loops through its values:
<?php
$fruits = array(“Apple”, “Banana”, “Orange”);
foreach ($fruits as $fruit) {
echo $fruit . “<br>”;
}
?>
In this script, we declared an array named “fruits” and assigned three values to it. We then used a “foreach” loop to loop through each value in the array and output it to the screen. The “<br>” tag is used to insert a line break between each value.
Conclusion
In this beginner’s guide, we covered the basics of PHP, including how to install it, set up a development environment, and write your first PHP script. We also learned about variables and arrays in PHP and how to work with them. This is just the tip of the iceberg when it comes to PHP, and there is much more to learn about the language. As you continue to learn, you’ll discover the vast range of features and functionality that PHP has to offer.
PHP is an excellent choice for beginners who want to learn web development because it has a relatively low learning curve compared to other languages. It is also widely used in the industry, which means there are many resources available for learning and troubleshooting.
If you’re interested in taking your PHP skills to the next level, there are many resources available online, including tutorials, forums, and documentation. You can also consider taking a PHP course or certification program to gain a more in-depth understanding of the language.
In conclusion, PHP is a powerful language for web development, and it’s relatively easy to get started. By following the steps outlined in this beginner’s guide, you can quickly set up your development environment and start writing your first PHP scripts. With time and practice, you’ll be able to build more complex web applications and contribute to the thriving PHP community.
The author of article is Jeff Vertes, the analyst of bestcasinosincanada.net